Here are four types of exclusive arguments in python. The arguments are passers to functions. These are Required arguments, Keyword arguments, Default arguments and Variable-length arguments.
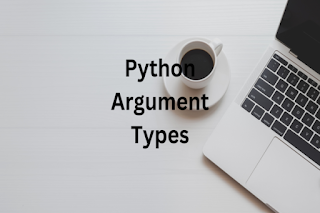
Here're 4 Important Arguments in Python
Argument#1: Required arguments
Positional arguments are known as required arguments and are passed to a function in the correct order. The arguments in the function call should match the number in the function definition.
Sample program:
def cal(a,b):
"""program to calculate sum of 2 numbers"""
sum=a+b
return sum
a=int(input("enter 1st number"))
b=int(input("enter 2nd number"))
print(cal(a,b))
Output
enter 1st number
10
enter 2nd number
22
32
** Process exited - Return Code: 0 **
Press Enter to exit terminal
Argument#2: Keyword arguments
A default argument is an argument that assumes a default value if a value is not provided in the function call for that argument.
The user may not want to provide values for some parameters for some functions, so you may want to make them optional and use default values. Default argument values are used to accomplish this. Add the assignment operator (=) followed by the default value to the parameter name in the function definition if you want to specify default argument values for parameters.
A constant value should be used for the default argument; it should be an immutable value by default.
Sample program:
def cal(a,b=20):
"""program to calculate sum of 2 numbers"""
sum=a+b
return sum
a=10
b=12
print(cal(a,b))
print(cal(a))
Output
22
30
** Process exited - Return Code: 0 **
Press Enter to exit terminal
Argument#3: Default arguments
Arguments related to function calls are known as keyword arguments. In function calls, parameter names are used to identify keyword arguments.
If you have some functions with many parameters and only want to specify some of them, you can call them keyword arguments.
Sample program:
def cal(a,b):
"""program to calculate sum of 2 numbers"""
sum=a+b
return sum
print(cal(a=12,b=24)) # 2 keyword argument
print(cal(b=24,a=12)) # 2 keyword argument(out of order)
print(cal(24,b=4)) # 1 positional, 1 keyword
print(cal(a=22,12))
Output
File "main.py", line 9
print(cal(a=22,12))
^
SyntaxError: positional argument follows keyword argument** Process exited - Return Code: 1 **
Press Enter to exit terminal
Argument#4: Variable-length arguments
They are also known as variable length arguments. In some cases, we do not know in advance how many arguments will be passed to a function. This kind of a situation can be handled in Python through function calls with arbitrary arguments. For this kind of an argument, we use an asterisk (*) before the parameter name in the function definition.
Sample program:
def display(arg1, *vartuple):
"Arbitrary arguments"
print ("Output is: ")
print (arg1)
for var in vartuple:
print (var)
return
display(34)
display(10,20,30,40)
Output
Output is:
34
Output is:
10
20
30
40
** Process exited - Return Code: 0 **
Press Enter to exit terminal
Comments
Post a Comment
Thanks for your message. We will get back you.